HTML - Images
Learn to add images to a webpage.
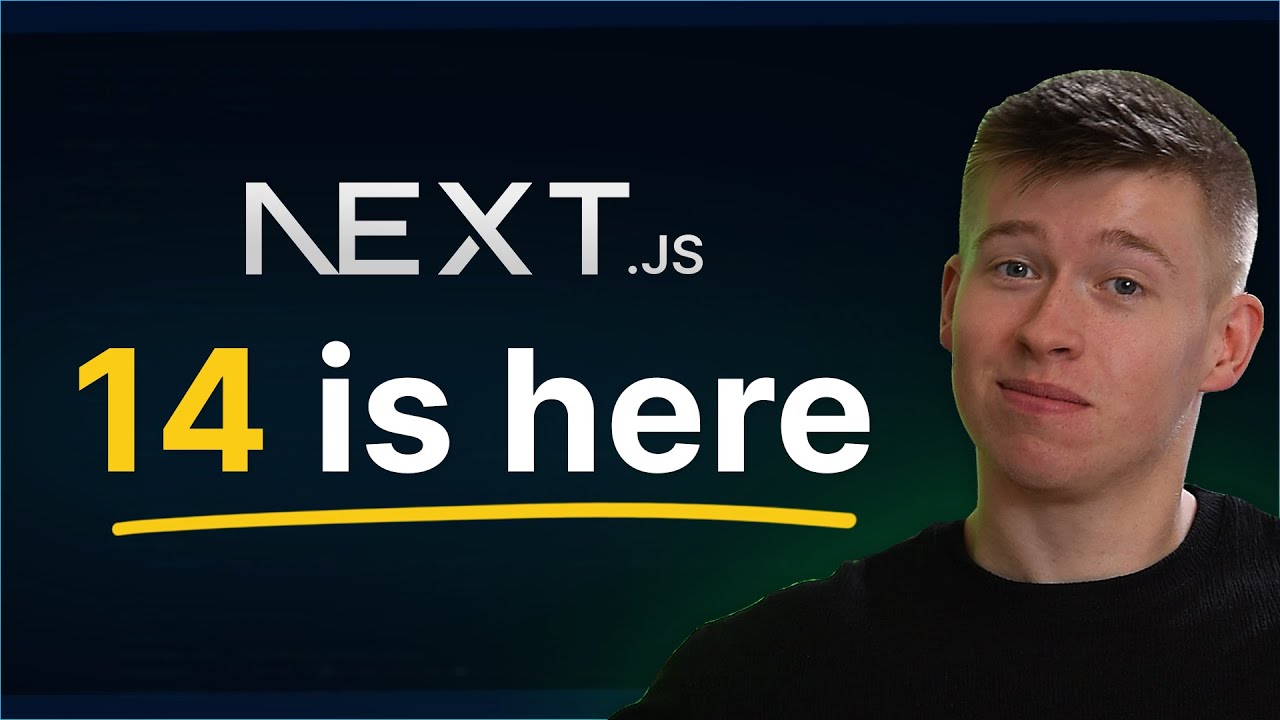
Image Basics
We can add images to our webpage using the img tag.
The img tag is a self-closing tag, meaning it does not require a closing tag.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Image Example</title>
</head>
<body>
<img>
</body>
</html>
We have to add a piece of additional information to the image tag to tell it what image we want to add.
We achieve this by adding the src attribute to the img tag.
An Attribute is simply an additional piece of information added to a tag.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Image Example</title>
</head>
<body>
<img src="">
</body>
</html>
Inside the quotation marks of the src attribute, we add the file path to the image.
If the image is in the same folder as our webpage then this is simply the filename of the image.
We have to also include the file extension of the image
If we had an image called logo.png saved in the same folder as our webpage we would write:
index.html
<!DOCTYPE html>
<html>
<head>
<title>Image Example</title>
</head>
<body>
<img src="logo.png">
</body>
</html>
File Paths
A file path is simply the location of a file on a computer.
There are two types of file paths we can use:
- Relative File Path
- Absolute File Path
Relative File Path
A relative file path is a file path that is relative to the current webpage.
This means that the file path is relative to the location of the webpage on the computer.
If the image is in the same folder as the webpage, we can simply write the filename of the image.
If the image is in a folder inside the same folder as the webpage, we can write the folder name followed by a forward slash and then the filename.
For example, If the logo.png file was in a folder called images we would write.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Image Example</title>
</head>
<body>
<img src="images/logo.png">
</body>
</html>
Common Issues
There are a few common issues that can occur when adding images to a webpage.
If your image is not loading then you should check the following.
File Name & Path
Have you spelled the name of the image correctly. This inlcudes capital letters and spaces.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Image Example</title>
</head>
<body>
<img src="logoo.png">
</body>
</html>
This also includes names of folders in the file path.
Image Extension
Have you included the correct file extension.
If the image is a png, have you accidently added .jpg or something else?
index.html
<!DOCTYPE html>
<html>
<head>
<title>Image Example</title>
</head>
<body>
<img src="logo.jpg">
</body>
</html>
Image Location
Is the image in the correct location?
You should check that the image is in the place you have said it is.
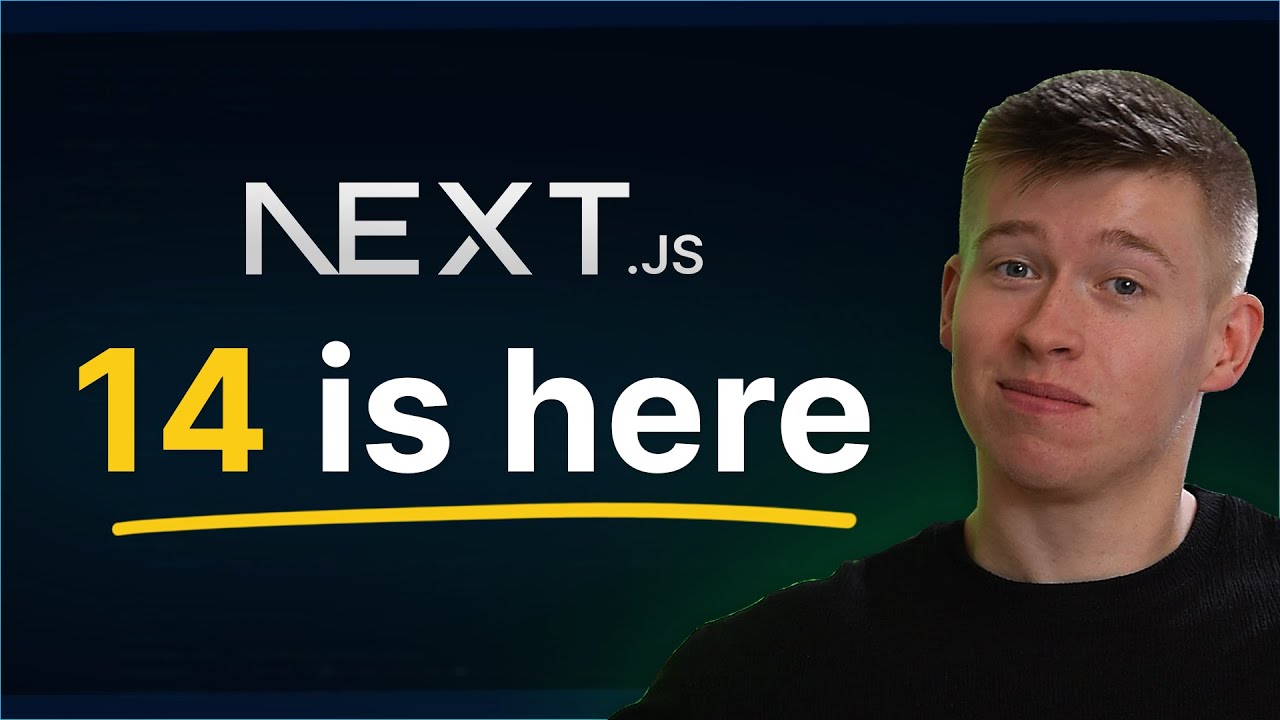