Variables
An introduction to variables
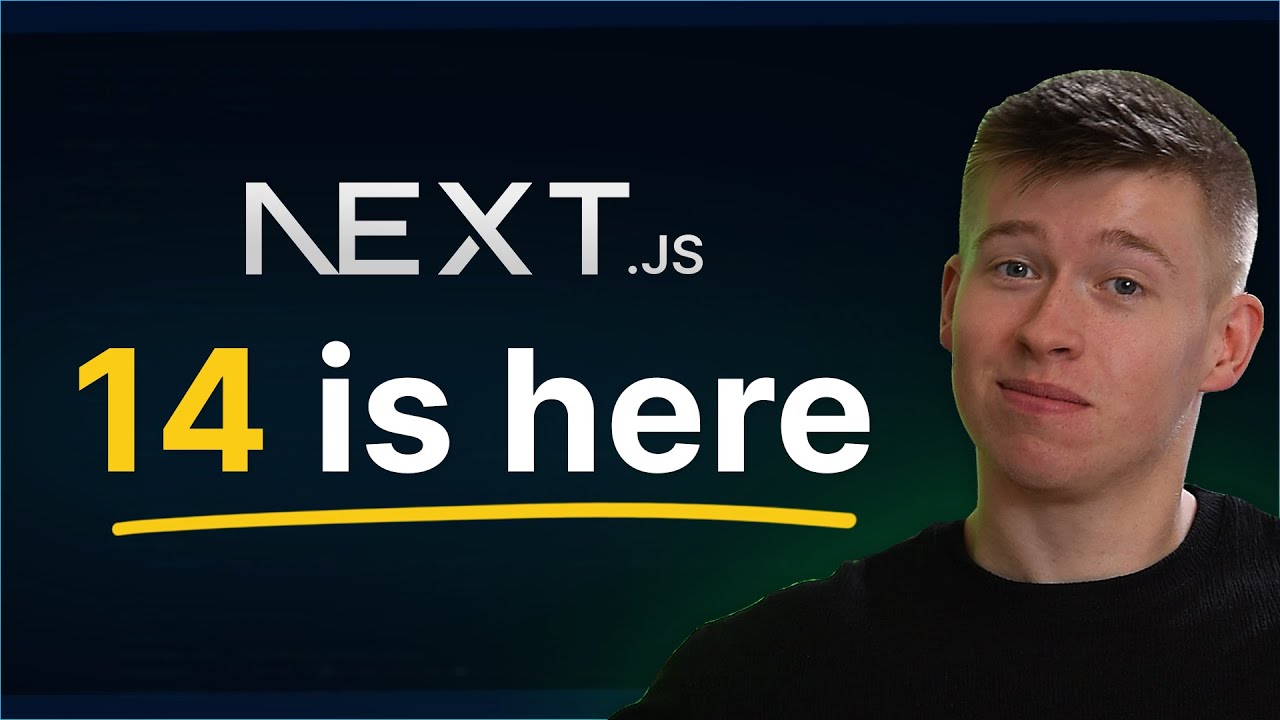
Introduction
One of the most important elements in programming is the variable.
Variables are used to store information that can be used later in the program.
In Python, we can store information in a variable and then display it on the screen using the print statement, or use it in some other part of our program.
You can think of a variable like a box that you can store a piece of data in.
You can then label the box with a name so that you can find it later.
In Python, we can create a variable and give it a name using the following syntax:
age = 16
Variables Names
In Python, we have to follow certain rules for naming variables.
Rules
- Variable names must start with a letter
- Other characters can be letters, numbers, or underscores
- Variable names cannot contain spaces
- You cannot use a reserved word (such as print)
To expand on the last point, there are several keywords in python that are reserved as they serve some some of purpose in the programming language.
There are several of these such as print, input, if, and, or, not, for, while, etc. All of these words are used in python as an instruction and therefore cannot be used as variable names. You will encounter more of these as you progress throught the course.
Variable names are also case-sensitive. This means that we must use the same uppercase and lowercase letters everytime we refer to a variable.
Naming Conventions
Python also has some naming conventions. These are guidelines that should be followed when naming your variables.
The rules discussed above must be followed otherwise the program will not work. However, it is simply good practice to follow naming conventions but our program will still function correctly if these are not followed.
- Variable names should be meaningful (student_number is better than sn)
- All letters should be lowercase
- Underscores should be used instead of spaces
Basic Usage
We can store any of our 5 data types in a variable.
name = "Lucy"
first_initial = "L"
age = 15
height 1.62
in_school = True
Each of these lines of code takes a piece of data and stores it in a variable that we can use later in our program.
We could display the contents of one of these variables by using the print statement.
name = "Lucy"
first_initial = "L"
age = 15
height 1.62
in_school = True
print(name)
Notice that there are no quotation marks around the print statement. When quotation marks are used, the actual word will be displayed. When no quotation marks are used, the contents of the variable with that name will be displayed.
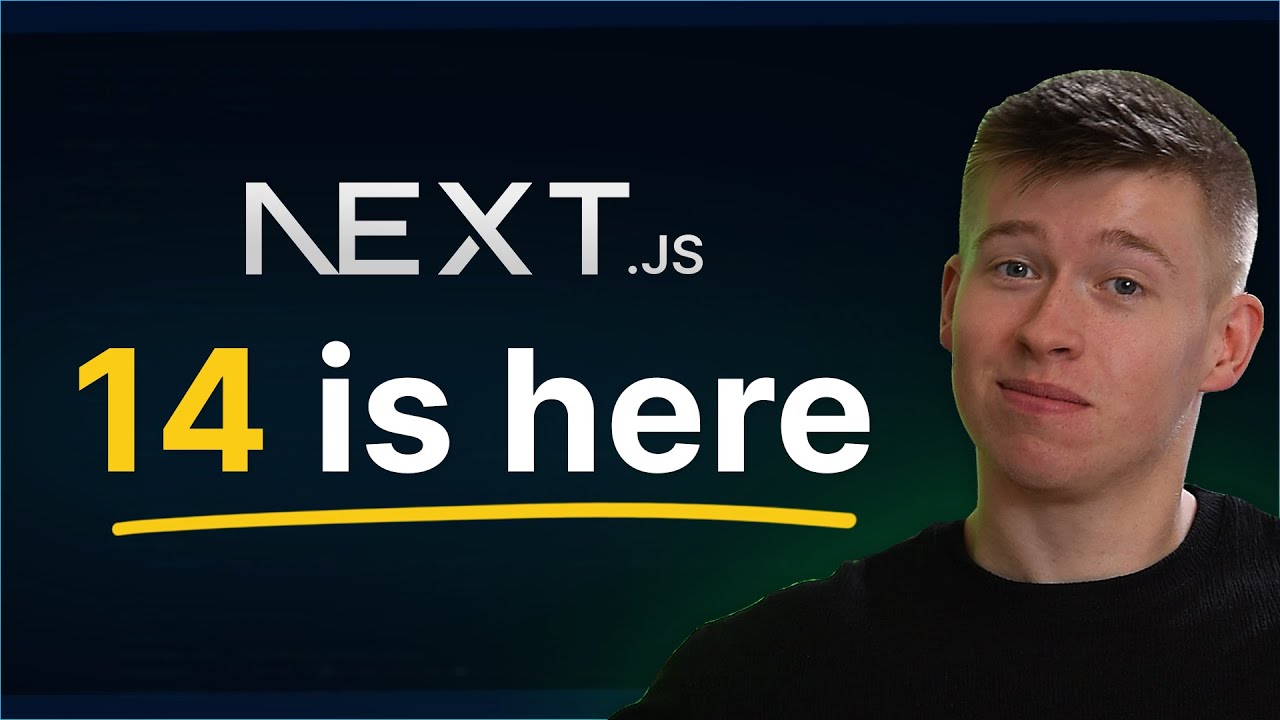