The Print Statement
Learn to display information using Python.
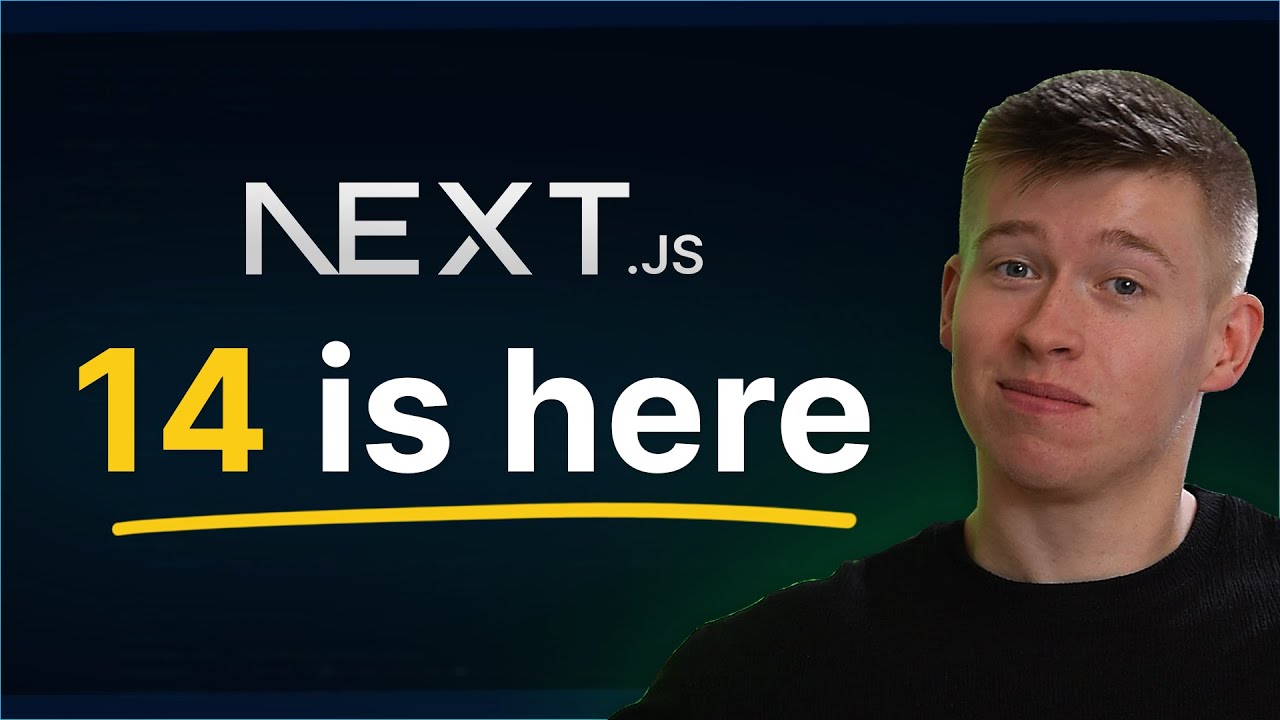
Introduction
One of the most basic things we can do in a programming language is to display something on the screen.
Using Python, we can achieve this using the print statement.
The Print Statement
Like all programming languages, Python contains command words that tell the program to do a certain thing. Print is one of these command words.
In Python, the print() command word tells the program to display something on the screen.
print()
The print() command word is followed by a pair of brackets. Inside these brackets, we type what we want to display on the screen.
This will usually be one of the 5 data types that we previously looked at.
Basic Usage
We can add any of the 5 data types that we previously used to our print statement to display them on the screen.
For example, to display the string "Hello, world!" we would write:
print("Hello, world!")
We could also use any of our other data types:
example.py
print("Hello, world!")
print("A")
print(42)
print(3.14)
print(True)
You can see from above that we have displayed the following data types:
- String
- Character
- Integer
- Real
- Boolean
We can use the print statement to display other information as well (such as variables). We will see how else this can be used as we progress through each tutorial.
Printing Variables & Strings
If you have not yet learned about variables go back and read the Variables section first!
We can join the contents of variables with strings within our print statements to create a more meaningful output
name = "Jackson"
age = 16
print(name, "is", age, "years old.")
Within our print statement, anytime we want to join two things together we use a comma.
The program above would produce the output Jackson is 16 years old.
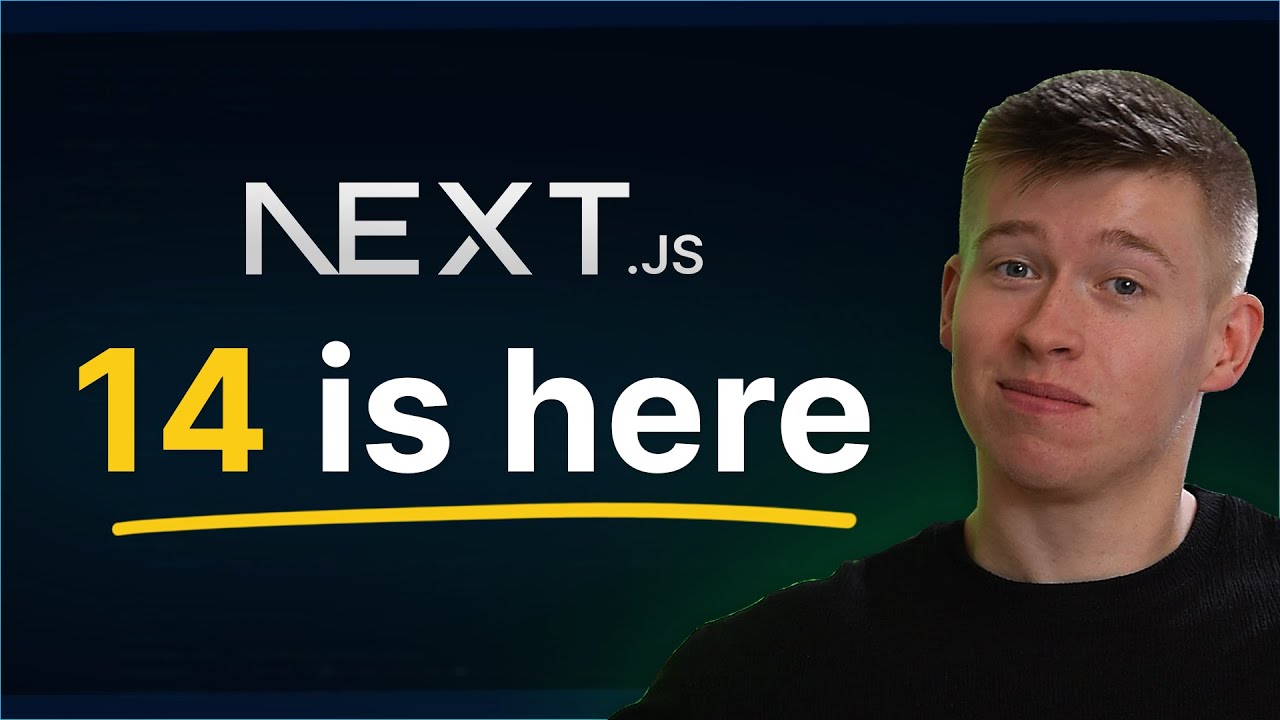