Procedures
Learn to use procedures in your program
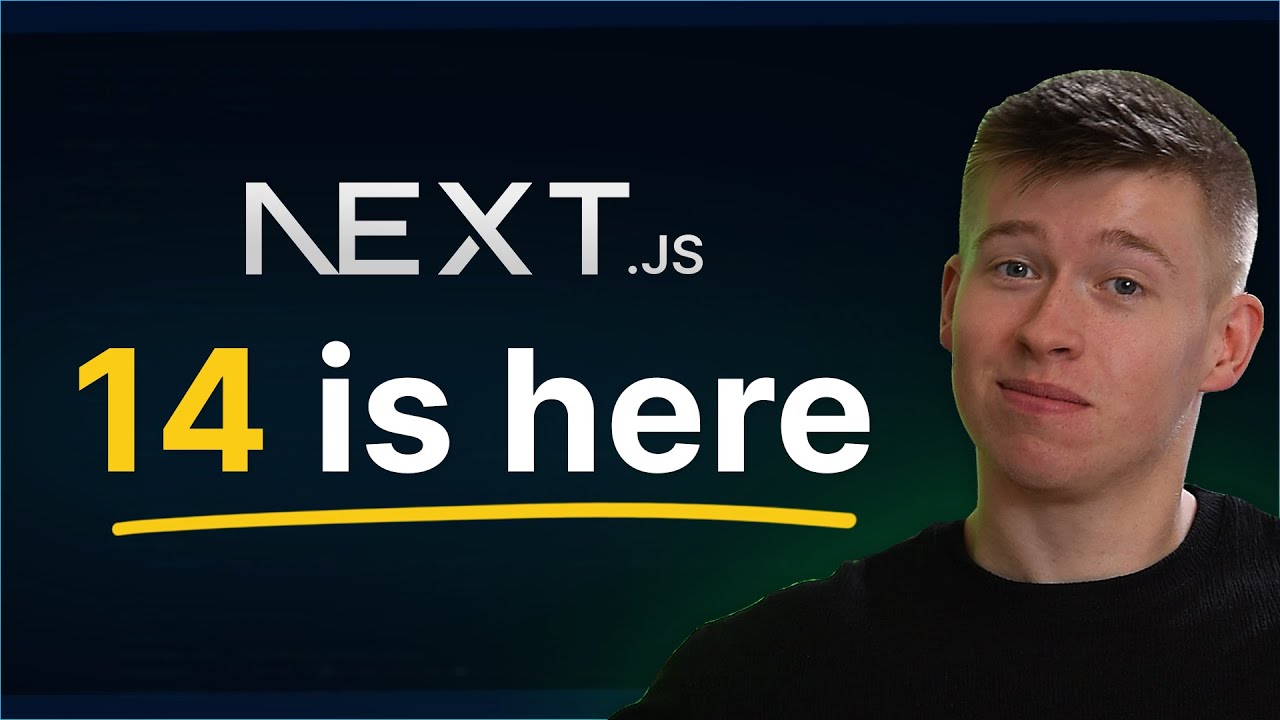
Introduction
One type of subprogram that we can create is called a procedure.
During this section you will learn how to create a procedure in your programs.
Procedures
The program below sets up a procedure that displays pupil details.
example.py
def display_details(name, mark):
print("Pupil:", name)
print("Mark:", mark)
The procedure is defined using the def keyword.
def
We then have to provide a name. This should be descriptive of what the procedure does.
def display_details():
When creating procedures we have to put brackets after the name. We then use a colon to signal the end of the line and the start of the block of code that will be contained within the procedure.
Inside the brackets we add parameters. These are variable names that will store any required information once the procedure is run. We separate each parameter with a comma.
def display_details(name, mark):
This is called the procedure declaration.
The next lines should be indented, as we would a conditional statement or a loop.
Any code that is indented will run when the procedure is run.
def display_details(name, mark):
print("Pupil:", name)
print("Mark:", mark)
If you were to type the code above into your IDE and run it, you'll notice that it doesn't do anything.
This is because procedures do not run automatically, they must be told to run. This is referred to as 'calling a procedure'.
To call the procedure above we would add the following to our program.
example.py
def display_details(name, mark):
print("Pupil:", name)
print("Mark:", mark)
display_details("Steven", 51)
We call the procedure by writing its name and giving it the required information.
display_details("Steven", 51)
Notice that when we called the procedure, we added the parameters in the same order as they were when we set up the procedure. Name first, mark second.
This is important as they will be passed to the procedure in that order.
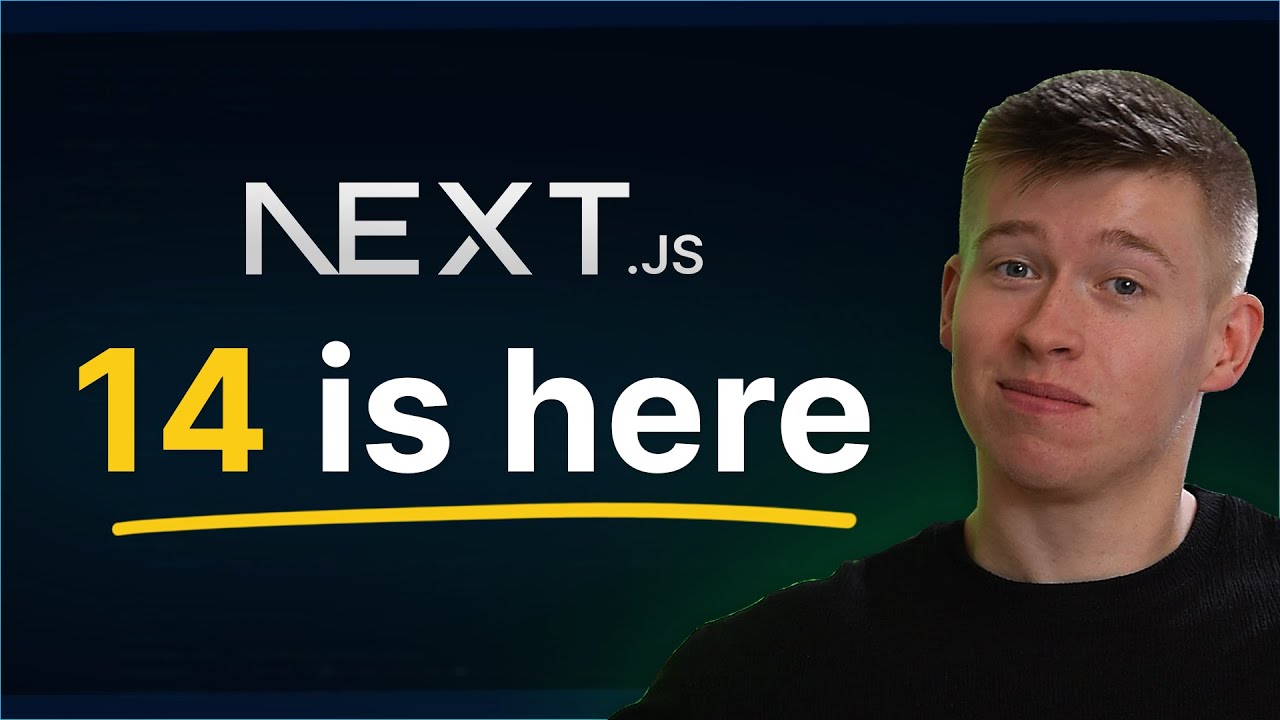