Functions
Learn to use functions in your program
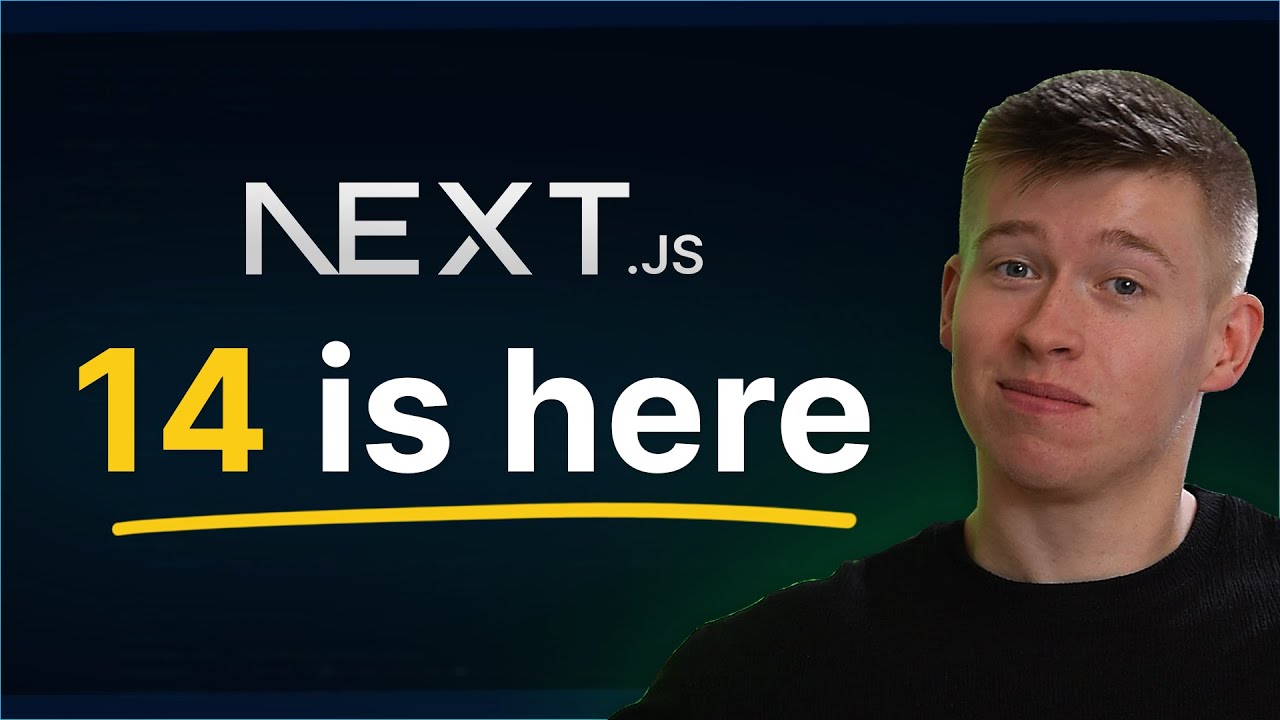
Introduction
Another type of subprogram that we can create is called a function.
During this section you will learn how to create and use functions in your programs.
Functions
The program below sets up a function that converts a temperature from Celsius to Fahrenheit and returns the result.
example.py
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 1.8) + 32
fahrenheit = round(fahrenheit, 2)
return fahrenheit
The function is defined using the def keyword.
def
We then have to provide a name. This should be descriptive of what the function does.
def celsius_to_fahrenheit():
When creating functions, we have to put brackets after the name. We then use a colon to signal the end of the line and the start of the block of code that will be contained within the function.
Inside the brackets, we add parameters. These are variable names that will store any required information once the function is run. We separate each parameter with a comma if there is more than one.
def celsius_to_fahrenheit(celsius):
This is called the function declaration.
The next lines should be indented, as we would a conditional statement or a loop.
Any code that is indented will run when the function is called.
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 1.8) + 32
fahrenheit = round(fahrenheit, 2)
return fahrenheit
Notice the return statement in the function. This is what makes functions different from procedures. A return statement allows a function to send back a result.
If you were to type the code above into your IDE and run it, you'll notice that it doesn't do anything by itself.
This is because functions do not run automatically; they must be called. This is referred to as 'calling a function'.
To call the function above and display its result, we would add the following to our program.
example.py
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 1.8) + 32
fahrenheit = round(fahrenheit, 2)
return fahrenheit
fahrenheit = celsius_to_fahrenheit(25)
print("Fahrenheit:", fahrenheit)
We call the function by writing its name and providing the required argument.
fahrenheit = celsius_to_fahrenheit(25)
The argument (25 in this case) is passed to the parameter celsius in the function declaration.
The return statement in the function instructs the program to pass data back to the main program.
We use a variable to store the data that is passed back.
In this case, The result of the function is stored in a variable called fahrenheit, which is then printed.
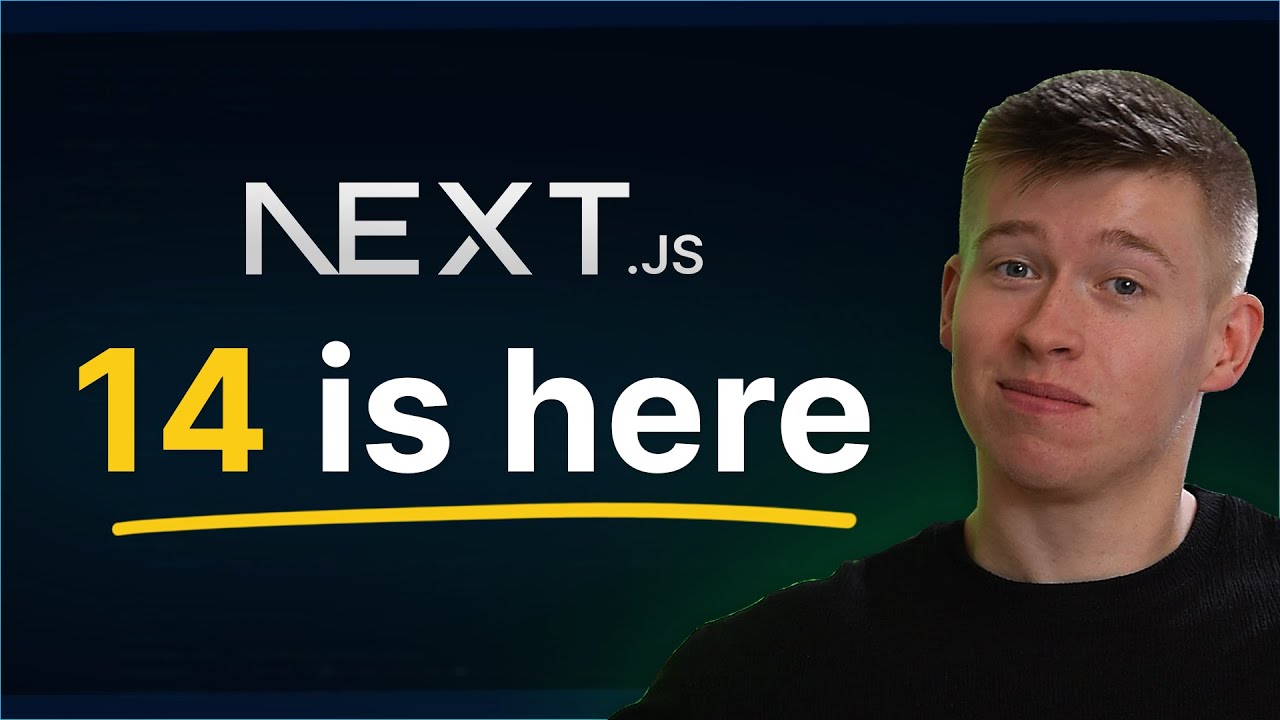